JavaScript is essential in web development for creating dynamic, interactive, and responsive user experiences. It powers everything from DOM manipulation and form validation to complex applications using frameworks like React and Angular. Learn how JavaScript enhances web functionality and usability.
Introduction
JavaScript is a critical technology in the web development landscape, widely recognized for its ability to create interactive and dynamic web experiences. From enhancing user interfaces to enabling real-time data updates and powering complex web applications, JavaScript serves as the backbone of modern web development. Its versatility extends to both client-side and server-side programming, making it an indispensable tool for developers aiming to build responsive, efficient, and user-friendly websites. This introduction explores the various ways JavaScript is utilized in web development, highlighting its role in shaping the interactive web as we know it today.
What is JavaScript Used For in Web Development?
JavaScript is a cornerstone technology in web development, integral to creating dynamic, interactive web experiences. Since its inception in 1995, it has evolved into a powerful language, underpinning everything from simple web page enhancements to complex server-side applications. Here’s an in-depth look at how JavaScript is used in web development:
1. Interactive User Interfaces
- Dynamic User Experiences: JavaScript is essential for crafting engaging user interfaces. It enables developers to build interactive elements like sliders, accordions, dropdown menus, and modal windows. By manipulating the Document Object Model (DOM), JavaScript can update the content and structure of web pages in real-time, enhancing user engagement without requiring page reloads.
- Event Handling: JavaScript’s ability to handle events such as clicks, mouse movements, and keyboard inputs allows for responsive and interactive web pages. Event listeners can be attached to various elements, triggering functions that provide immediate feedback to user actions.
2. Form Validation
- Client-Side Validation: JavaScript is widely used for form validation before data is submitted to the server. It can check for required fields, validate email addresses, and ensure that passwords meet specified criteria. This immediate feedback improves user experience by allowing users to correct errors in real-time, reducing server load and minimizing the need for additional server-side validation.
- Regular Expressions: JavaScript supports regular expressions, which can be used for sophisticated pattern matching in form validation. This allows developers to implement complex validation rules efficiently.
3. Dynamic Content Updates
- AJAX (Asynchronous JavaScript and XML): AJAX is a technique that uses JavaScript to send and receive data asynchronously without refreshing the web page. This enables web applications to fetch data from the server in the background and update the user interface dynamically, leading to faster and more seamless user experiences. For example, infinite scrolling, where new content loads as the user scrolls, is powered by AJAX.
- Fetch API: Modern JavaScript includes the Fetch API, a more flexible and powerful way to make asynchronous requests to servers compared to the older XMLHttpRequest object. It simplifies the process of fetching resources and handling responses, making it easier to work with APIs and dynamic data.
4. Animations and Effects
- CSS Animations and Transitions: JavaScript can be used to control and trigger CSS animations and transitions. By dynamically applying CSS classes, developers can create sophisticated visual effects that enhance the user experience.
- JavaScript Animation Libraries: Libraries such as GreenSock Animation Platform (GSAP) and Anime.js provide advanced tools for creating complex animations. These libraries offer fine-grained control over animations, enabling developers to create engaging and visually appealing web pages.
5. Client-Side Storage
- Local Storage: JavaScript’s local storage API allows web applications to store data locally within the user’s browser. This can be used to save user preferences, store session data, and cache resources for offline access. Data stored in local storage persists across browser sessions, making it ideal for saving settings and state information.
- Session Storage: Similar to local storage, session storage is used to store data for the duration of the page session. It is useful for temporary data that should not persist after the user closes the tab or browser.
- IndexedDB: For more complex storage needs, JavaScript provides IndexedDB, a low-level API for storing large amounts of structured data. It allows developers to build applications that can work offline and synchronize data with a server once an internet connection is available.
6. Single Page Applications (SPAs)
- Frameworks and Libraries: JavaScript frameworks like React, Angular, and Vue.js are fundamental to building SPAs. SPAs load a single HTML page and dynamically update the content as the user interacts with the application. This approach provides a smoother, faster user experience compared to traditional multi-page applications.
- Component-Based Architecture: Frameworks such as React use a component-based architecture, where the UI is divided into reusable components. Each component manages its own state and lifecycle, making it easier to develop, test, and maintain complex applications.
7. Server-Side Development
- Node.js: Node.js is a JavaScript runtime built on Chrome’s V8 JavaScript engine that allows developers to run JavaScript on the server. With Node.js, developers can build scalable and high-performance server-side applications using JavaScript. It is particularly well-suited for real-time applications like chat apps and online gaming due to its non-blocking, event-driven architecture.
- Server-Side Frameworks: Express.js is a popular Node.js framework that simplifies the development of web applications and APIs. It provides robust tools for routing, middleware integration, and HTTP request handling.
8. APIs and JSON Parsing
- RESTful APIs: JavaScript is commonly used to interact with RESTful APIs, enabling web applications to communicate with external services. This allows developers to integrate third-party data, services, and functionalities into their applications.
- JSON (JavaScript Object Notation): JSON is a lightweight data-interchange format that is easy to read and write for humans and easy to parse and generate for machines. JavaScript’s native support for JSON makes it the preferred format for data exchange between the client and server.
9. Real-Time Applications
- WebSockets: JavaScript supports WebSockets, a protocol that enables full-duplex communication channels over a single TCP connection. This is ideal for real-time applications such as live chat, online gaming, and collaborative tools, where timely data updates are crucial.
- Socket.IO: Socket.IO is a library that abstracts WebSocket communication, providing a simple and reliable API for building real-time applications. It includes features like automatic reconnection, multiplexing, and room support.
10. Progressive Web Apps (PWAs)
- Service Workers: Service workers are a JavaScript feature that enables offline capabilities, background synchronization, and push notifications in web applications. They act as a proxy between the web application and the network, allowing developers to cache resources and provide a seamless offline experience.
- App Shell Model: The app shell model is a design approach used in PWAs where the basic structure of the app is loaded and cached initially, and content is loaded dynamically as needed. This approach, enabled by JavaScript, ensures fast load times and a smooth user experience.
DOM Manipulation
DOM (Document Object Model) manipulation is a fundamental concept in web development that allows developers to interact with and modify the structure, style, and content of a web page dynamically. This is achieved using JavaScript, which provides the tools and methods necessary to access and change the elements within the HTML document. Understanding and effectively using DOM manipulation is crucial for creating interactive and responsive web applications. Here’s a detailed look at DOM manipulation and its various aspects:
What is the DOM?
The Document Object Model (DOM) is a programming interface for web documents. It represents the structure of an HTML or XML document as a tree of nodes, where each node corresponds to a part of the document, such as an element, attribute, or piece of text. The DOM allows developers to access and manipulate these nodes using scripting languages like JavaScript.
Key Methods and Properties for DOM Manipulation
Selecting Elements: To manipulate the DOM, you first need to select the elements you want to work with. JavaScript provides several methods for selecting elements:
- document.getElementById(id): Selects an element by its ID.
- document.getElementsByClassName(className): Selects all elements with a given class.
- document.getElementsByTagName(tagName): Selects all elements with a given tag name.
- document.querySelector(selector): Selects the first element that matches a CSS selector.
- document.querySelectorAll(selector): Selects all elements that match a CSS selector.
Example: javascript
var element = document.getElementById(‘myElement’);
var elements = document.getElementsByClassName(‘myClass’);
var firstDiv = document.querySelector(‘div’);
var allDivs = document.querySelectorAll(‘div’);
Creating and Inserting Elements: JavaScript allows you to create new elements and insert them into the DOM.
- document.createElement(tagName): Creates a new element.
- element.appendChild(child): Appends a child element to a parent element.
- element.insertBefore(newElement, referenceElement): Inserts a new element before a reference element.
Example: javascript
var newDiv = document.createElement(‘div’);
newDiv.textContent = ‘Hello, World!’;
document.body.appendChild(newDiv);
Modifying Elements: Once you have selected an element, you can modify its attributes, styles, and content.
- element.setAttribute(name, value): Sets the value of an attribute.
- element.removeAttribute(name): Removes an attribute.
- element.style.property = value: Sets a CSS property.
- element.innerHTML: Sets the HTML content inside an element.
- element.textContent: Sets the text content inside an element.
Example: javascript
var element = document.getElementById(‘myElement’);
element.setAttribute(‘class’, ‘newClass’);
element.style.backgroundColor = ‘yellow’;
element.innerHTML = ‘<p>New content</p>’;
Removing Elements: Elements can be removed from the DOM using the following methods:
- element.removeChild(child): Removes a child element from a parent element.
- element.remove(): Removes the element itself.
Example:
javascript
Copy code
var parent = document.getElementById(‘parent’);
var child = document.getElementById(‘child’);
parent.removeChild(child);
var element = document.getElementById(‘element’);
element.remove();
Event Handling: DOM manipulation often involves responding to user interactions through event handling. JavaScript provides methods to attach event listeners to elements.
- element.addEventListener(event, function): Attaches an event listener to an element.
- element.removeEventListener(event, function): Removes an event listener from an element.
Example: javascript
var button = document.getElementById(‘myButton’);
button.addEventListener(‘click’, function() {
alert(‘Button clicked!’);
});
Practical Applications of DOM Manipulation
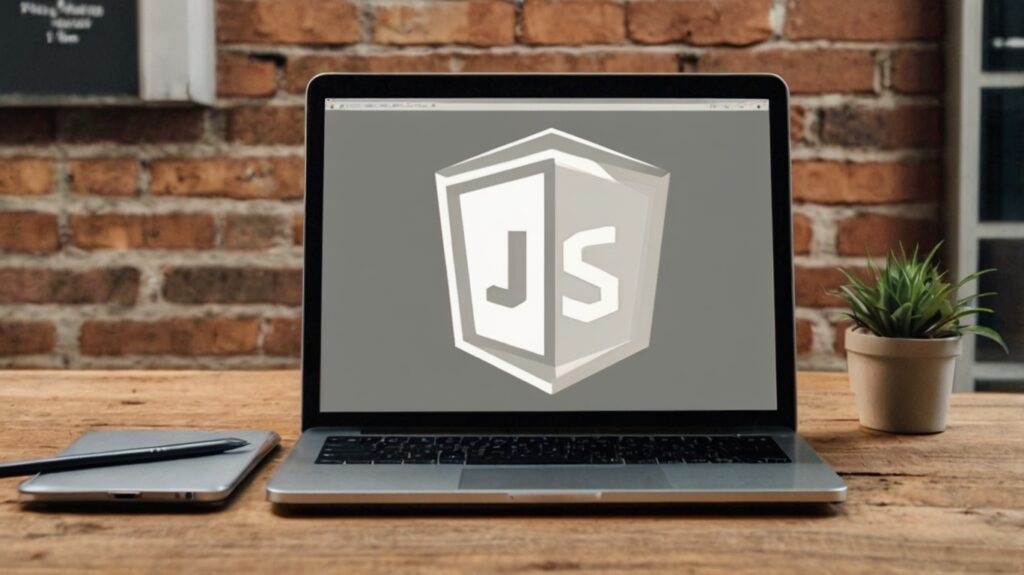
- Dynamic Content Updates: DOM manipulation allows web pages to update content dynamically in response to user actions or external data. For example, an e-commerce site can use DOM manipulation to update the shopping cart contents without reloading the page.
- Form Validation and Submission: JavaScript can validate form inputs in real-time, providing immediate feedback to users. It can also modify the form’s behavior upon submission, such as showing a loading spinner or displaying success/error messages.
- Interactive User Interfaces: Features like modals, tabs, carousels, and tooltips rely on DOM manipulation to show or hide elements and provide a smooth user experience.
- Animations and Effects: JavaScript can create animations by changing an element’s style properties over time. Libraries like GSAP and jQuery simplify the process of animating DOM elements.
- Single Page Applications (SPAs): SPAs use DOM manipulation to load new content and update the view without refreshing the entire page. This creates a fast, responsive experience similar to native applications.
Performance Considerations
While DOM manipulation is powerful, it can also impact performance if not used judiciously. Here are some tips for efficient DOM manipulation:
Minimize Reflows and Repaints: Changes to the DOM can cause reflows (layout recalculations) and repaints (visual updates), which are performance-intensive. Batch DOM updates and minimize changes to the layout to improve performance.
Use Document Fragments: When adding multiple elements to the DOM, use a DocumentFragment to batch updates and insert the fragment into the DOM in a single operation.
Example: javascript
var fragment = document.createDocumentFragment();
for (var i = 0; i < 100; i++) {
var div = document.createElement(‘div’);
div.textContent = ‘Item ‘ + i;
fragment.appendChild(div);
}
document.body.appendChild(fragment);
Debounce and Throttle Events: For events that fire frequently (like scroll or resize), use debouncing or throttling techniques to limit the number of times the event handler executes.
Example:javascript
function debounce(func, wait) {
let timeout;
return function(…args) {
clearTimeout(timeout);
timeout = setTimeout(() => func.apply(this, args), wait);
};
}
window.addEventListener(‘resize’, debounce(function() {
console.log(‘Resized’);
}, 250));
JavaScript Libraries and Frameworks
JavaScript libraries and frameworks play a pivotal role in modern web development, offering pre-written code and tools that simplify the development process and enhance productivity. These libraries and frameworks provide a structured approach to building complex web applications, allowing developers to focus on writing efficient and maintainable code. Here’s an in-depth look at some of the most popular JavaScript libraries and frameworks, their features, and their use cases:
JavaScript Libraries
1. jQuery
jQuery is one of the most widely used JavaScript libraries, designed to simplify HTML DOM manipulation, event handling, animation, and AJAX interactions.
- Ease of Use: jQuery’s syntax is concise and easy to learn, making it accessible for beginners.
- Cross-Browser Compatibility: jQuery handles many cross-browser issues, ensuring consistent behavior across different browsers.
- Rich Plugin Ecosystem: There are thousands of plugins available for extending jQuery’s functionality.
Use Cases:
- Simplifying complex DOM manipulations.
- Creating animations and effects.
- Handling AJAX requests.
Example
$(document).ready(function(){
$(“button”).click(function(){
$(“p”).hide();
});
});
2. Lodash
Lodash is a utility library that provides helpful functions for common programming tasks, making JavaScript development easier and more efficient.
- Utility Functions: Lodash offers a wide range of functions for manipulating arrays, objects, strings, and more.
- Performance: Lodash is optimized for performance and can handle large datasets efficiently.
- Modular: Lodash can be imported as individual modules, reducing the bundle size.
Use Cases:
- Data manipulation and transformation.
- Functional programming.
- Improving code readability and maintainability.
Example:
var users = [
{ ‘user’: ‘fred’, ‘age’: 48 },
{ ‘user’: ‘barney’, ‘age’: 36 },
{ ‘user’: ‘fred’, ‘age’: 40 },
{ ‘user’: ‘barney’, ‘age’: 34 }
];
var sortedUsers = _.sortBy(users, [‘user’, ‘age’]);
console.log(sortedUsers);
3. D3.js
D3.js is a powerful library for creating data visualizations using web standards like SVG, Canvas, and HTML.
- Data-Driven: D3.js binds data to DOM elements, allowing for dynamic and interactive visualizations.
- Highly Customizable: Offers fine-grained control over the appearance and behavior of visual elements.
- Scalable: Suitable for both small-scale visualizations and complex, large-scale data projects.
Use Cases:
- Creating interactive charts and graphs.
- Data-driven animations.
- Building complex dashboards and data visualizations.
Example:
var data = [30, 86, 168, 281, 303, 365];
d3.select(“.chart”)
.selectAll(“div”)
.data(data)
.enter().append(“div”)
.style(“width”, function(d) { return d + “px”; })
.text(function(d) { return d; });
JavaScript Frameworks
1. React
React is a popular JavaScript framework developed by Facebook, designed for building user interfaces, particularly single-page applications.
- Component-Based: React’s component-based architecture promotes reusability and modularity.
- Virtual DOM: React’s virtual DOM improves performance by minimizing direct DOM manipulations.
- Strong Community Support: React has a large and active community, providing a wealth of resources and third-party libraries.
Use Cases:
- Building dynamic and responsive user interfaces.
- Developing single-page applications (SPAs).
- Creating reusable UI components.
Example:
import React from ‘react’;
function App() {
return (
<div className=”App”>
<h1>Hello, world!</h1>
</div>
);
}
export default App;
2. Angular
Angular is a comprehensive framework developed by Google for building robust, scalable web applications.
- MVC Architecture: Angular follows the Model-View-Controller architecture, promoting separation of concerns.
- Two-Way Data Binding: Automatically synchronizes data between the model and view components.
- Dependency Injection: Angular’s built-in dependency injection simplifies testing and enhances modularity.
Use Cases:
- Developing enterprise-scale web applications.
- Building complex SPAs with rich user interfaces.
- Creating highly maintainable and testable codebases.
Example:
import { Component } from ‘@angular/core’;
@Component({
selector: ‘app-root’,
template: `<h1>Hello, world!</h1>`
})
export class AppComponent {}
3. Vue.js
Vue.js is a progressive JavaScript framework for building user interfaces, designed to be incrementally adoptable.
- Easy to Learn: Vue’s syntax is simple and intuitive, making it easy to pick up for beginners.
- Flexible: Can be used for small projects or integrated into larger ones with other libraries.
- Reactive Data Binding: Vue’s reactivity system ensures the UI automatically updates when the data changes.
Use Cases:
- Building small to medium-sized web applications.
- Adding interactivity to existing projects.
- Creating SPAs and complex UI components.
Example:
<template>
<div id=”app”>
<h1>{{ message }}</h1>
</div>
</template>
<script>
export default {
data() {
return {
message: ‘Hello, world!’
}
}
}
</script>
Choosing the Right Library or Framework
Choosing the right library or framework depends on the specific needs and goals of your project. Here are some factors to consider:
- Project Complexity: For simple projects, lightweight libraries like jQuery may suffice. For more complex applications, frameworks like React, Angular, or Vue.js provide the necessary structure and features.
- Performance: Consider the performance implications of your choice. Libraries like Lodash are optimized for performance, while frameworks like React use virtual DOM to enhance efficiency.
- Community and Ecosystem: A strong community and a rich ecosystem of plugins and extensions can significantly reduce development time and effort.
- Learning Curve: Assess the learning curve associated with each library or framework. Vue.js, for example, is known for its gentle learning curve, while Angular may require more time to master due to its complexity.
FAQ’s
SPAs are web applications that load a single HTML page and dynamically update the content as the user interacts with the app. JavaScript frameworks like React, Angular, and Vue.js are essential for building SPAs, enabling a smoother and faster user experience.
JavaScript libraries such as D3.js enable developers to create interactive and dynamic data visualizations. These visualizations can include charts, graphs, and maps that update in real-time based on user interactions or data changes.
Yes, JavaScript can create animations and visual effects on web pages. Libraries like GSAP (GreenSock Animation Platform) and frameworks like jQuery simplify the process of implementing complex animations and transitions.
PWAs are web applications that provide a native app-like experience on the web. JavaScript enables key PWA features such as offline functionality, background sync, and push notifications, making web apps more reliable and engaging.
JavaScript can make asynchronous HTTP requests using AJAX (Asynchronous JavaScript and XML). This allows web pages to fetch data from the server in the background and update the content dynamically without reloading the page, resulting in a smoother user experience.
Conclusion
JavaScript is a cornerstone of modern web development, enabling dynamic, interactive, and responsive user experiences. It allows developers to manipulate the DOM, validate forms, handle events, and make asynchronous requests, all of which enhance the functionality and usability of websites. With powerful frameworks and libraries like React, Angular, and Node.js, JavaScript facilitates the creation of complex applications, including single-page applications (SPAs) and progressive web apps (PWAs). Its versatility and widespread adoption make JavaScript essential for both client-side and server-side development, driving the evolution of the web.